- Published on
How to deploy your website with S3 and Cloudfront with AWS CDK Python
- Authors
- Name
- Katherine Moreno
Introduction
Learn how to deploy your website with S3 and Cloudfront . This is what we are going to build 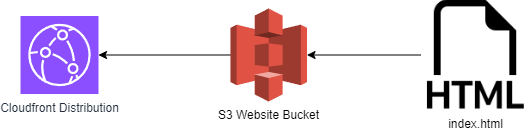
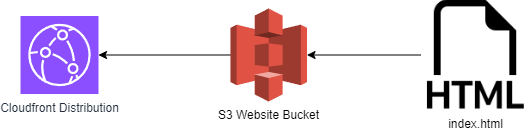
How I did it
I created the folder 'website' and include a simple html called index.html
<!DOCTYPE html>
<html>
<head>
<title>
My static website made with S3 and Cloudfront
</title>
</head>
<body>
Hello World!
</body>
</html>
and In our Stack I had to define the following:
from aws_cdk import (
CfnOutput,
Stack,
aws_s3 as s3,
aws_s3_deployment as s3deploy,
)
from aws_cdk.aws_cloudfront import BehaviorOptions, Distribution, OriginAccessIdentity
from aws_cdk.aws_cloudfront_origins import S3Origin
from constructs import Construct
import os
class MyStack(Stack):
def __init__(self, scope: Construct, construct_id: str, **kwargs) -> None:
super().__init__(scope, construct_id, **kwargs)
# Create a bucket to host the website
website_bucket = s3.Bucket(
self, "website_bucket", access_control=s3.BucketAccessControl.PRIVATE
)
# Verify that the folder which contains the index.html exists
website_dir = os.path.join(os.path.dirname(__file__), "website")
if not os.path.isdir(website_dir):
raise FileNotFoundError(f"The directory does {website_dir} not exist")
qr_origin_access_identity = OriginAccessIdentity(self, "OriginAccessIdentity")
website_bucket.grant_read(qr_origin_access_identity)
# Create the CF distribtuion
cf_distribution = Distribution(
self,
"Distribution",
default_root_object="index.html",
default_behavior=BehaviorOptions(
origin=S3Origin(
website_bucket, origin_access_identity=qr_origin_access_identity
)
),
)
s3deploy.BucketDeployment(
self,
"DeployWebsite",
sources=[s3deploy.Source.asset(website_dir)],
destination_bucket=website_bucket,
distribution=cf_distribution,
distribution_paths=["/*"], # This is for invalidation of the cache
)
# This makes to print in the console log the domain of the distribution
CfnOutput(self, "DomainName", value=cf_distribution.domain_name)
Epilogue
Enjoy your website!